Exploration with python in rhinoceros
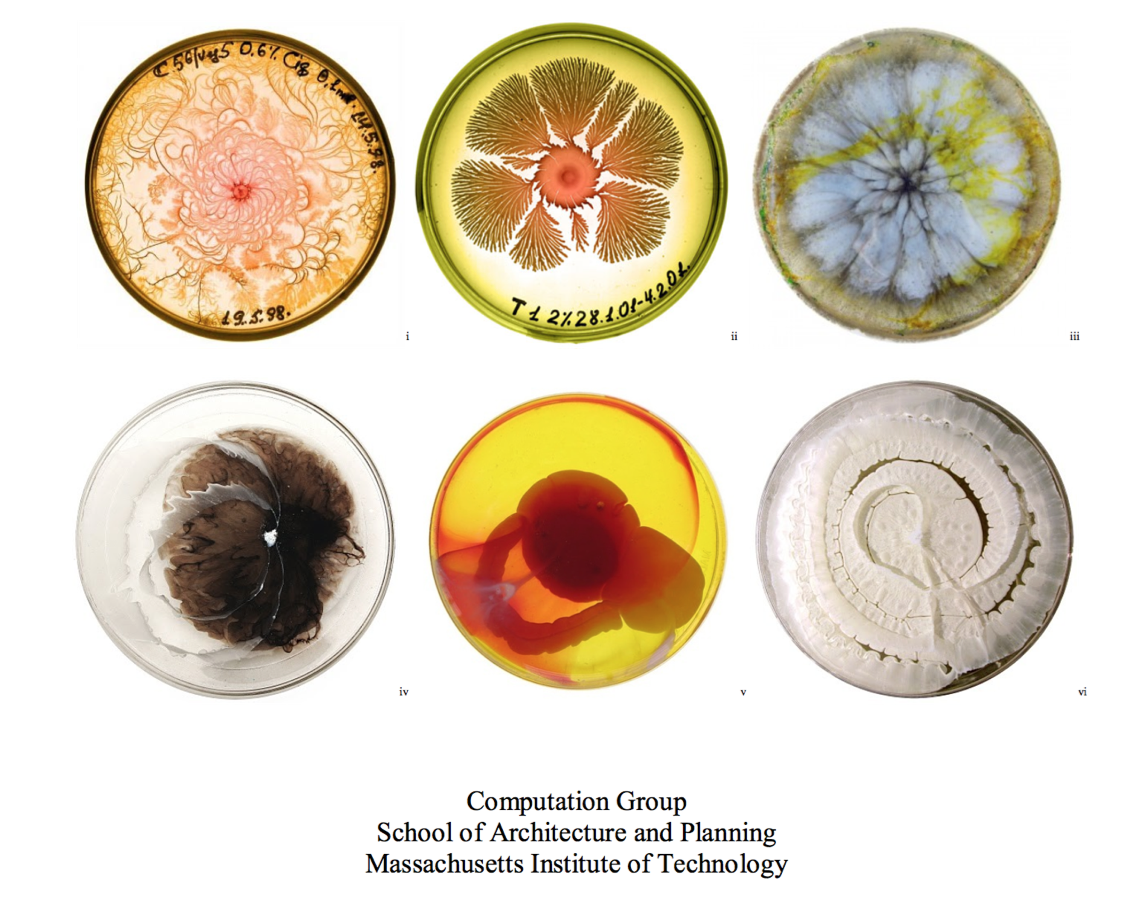
This work was created by looking at the inherent patterns and structures found in nature. Researching the makeup of organisms brings to light the complexities of recursive algorithms, L systems, and cellular automatae that are all found in the natural world. Bacteria is an element found in nature that is composed of a parametric structure. An algorithm based design process was used to look further into the structure of bacteria and various scripting tools were used to replicate the structure through visual examples. Python language was the most appropriate tool to generate the digital geometry in this example, as well as Rhino and various other 3D modeling software.
The code below generates curves that propagates and scale themselves to generate the recursive structure. The user has to generate the curve guide and the script will autamitcally scale and rotate.
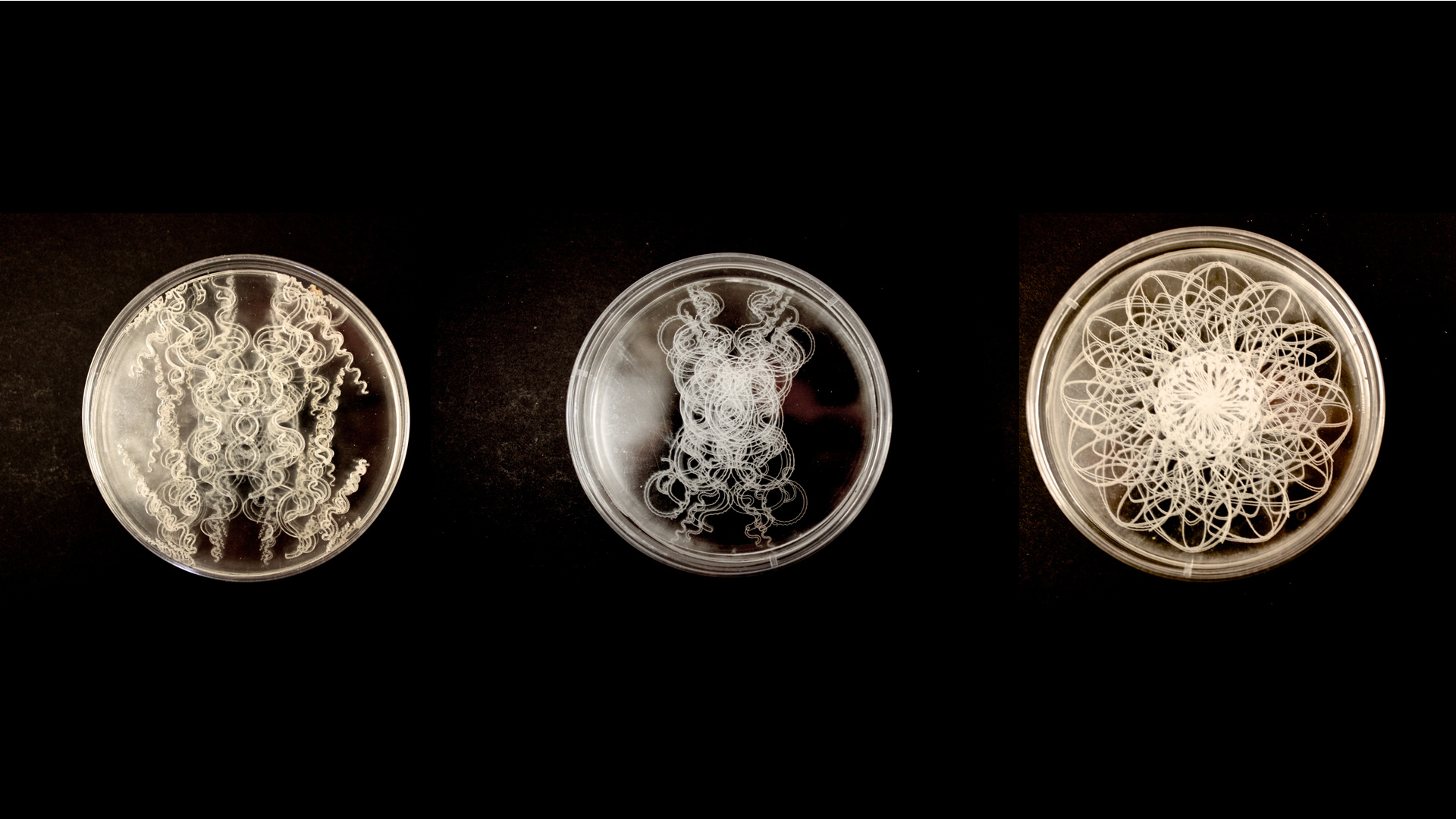
The first challenge was to create a 2D visual example of the cell structure; once it was recreated digitally, a laser cutter was used to etch multiple sheets of acrylic which were then laminated within a petri dish to create a layered visual example of the structure.
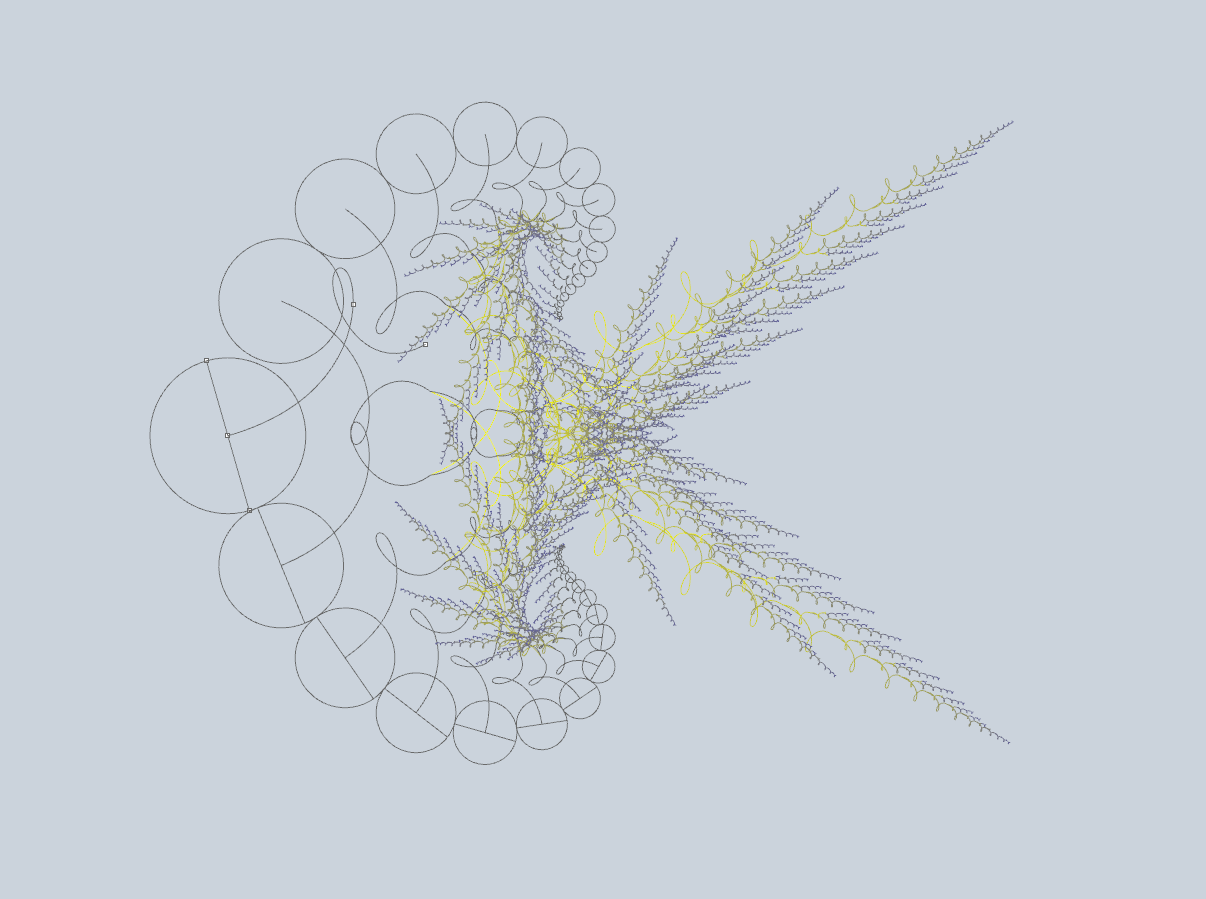
import rhinoscriptsyntax as rs def InitialCrv(): pts = rs.GetPoints('pick pts',1) # Pick points to generate curve curveID = rs.AddCurve(pts) return curveID def dividedSpline (input): # Divides the curve into gements NewCurve = rs.DivideCurve(input, 50, True,True) return NewCurve def bodyShape (Spl): #This function creates the body for i in range (0,51): body = rs.AddCircle(Spl[i],1.75) half = rs.DivideCurve(body, 2, True, True) return half def VectorFind (input): points = rs.DivideCurve(input, 50, True,True) vectorList = [] vectorList2 = [] param = 0 for i in points : # #print reverseVector param += 0.02 vectorList.append (unitizedVector) vectorList2.append(reverseVector) return [vectorList, vectorList2] def OffsetPt ( vectors,points): newPts = [] for i in range(0,51) : pt = rs.AddPoint(points[i]) vectors[i] = rs.VectorUnitize(vectors[i]) vectors[i]*=5 endPts = rs.MoveObject(pt, vectors[i]) newPts.append(endPts) newPts1 = [] for i in range(0,51) : pt1 = rs.AddPoint(points[i]) vectors[i] = rs.VectorUnitize(vectors[i]) vectors[i]*= -5 newPts1.append (pt1) for i in range(0,51): rs.AddLine(newPts[i], newPts1[i]) # result = [ newPts, newPts1, endPts] return [ newPts, newPts1, endPts ] def makeNewWorm(): output = InitialCrv() pts = dividedSpline (output) vect = VectorFind(output) rightPt = OffsetPt(vect[0], pts) leftPt = OffsetPt(vect[1], pts) bod = bodyShape (pts) newBody (bod,rightPt,leftPt) makeNewWorm()